LinearLayout
1) 개요
- 부모 컨테이너와의 상대적 위치 또는 다른 뷰와의 상대적 위치로 배치하는 레이아웃
2) 부모 컨테이너와의 상대적 위치를 이용
- layout_alignParent(Top, Bottom, Left, Right) : 부모 컨테이너의 위, 아래, 왼쪽, 오른쪽에 맞춰 배치
- layout_center(Horizontal, Vertical, Parent) : 부모 컨테이너의 수평 중앙, 수직 중앙, 정중앙에 배치
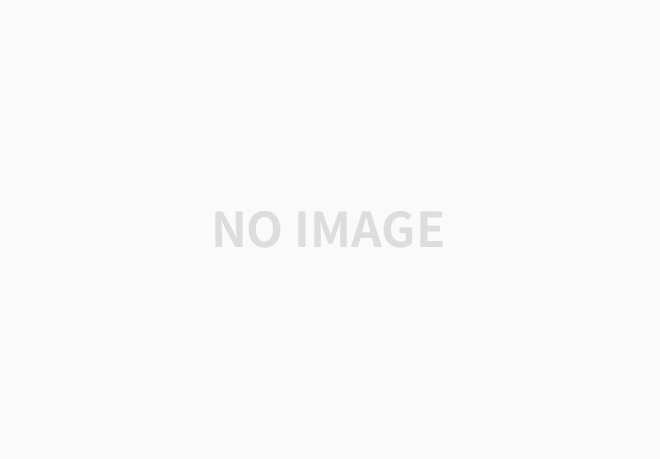
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- 1번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_alignParentTop="true"
android:text="1"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 2번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_centerHorizontal="true"
android:text="2"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 3번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_alignParentRight="true"
android:text="3"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 4번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_centerVertical="true"
android:text="4"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 5번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_centerInParent="true"
android:text="5"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 6번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_alignParentRight="true"
android:layout_centerVertical="true"
android:text="6"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 7번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_alignParentBottom="true"
android:text="7"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 8번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_centerHorizontal="true"
android:layout_alignParentBottom="true"
android:text="8"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 9번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_alignParentRight="true"
android:layout_alignParentBottom="true"
android:text="9"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
</RelativeLayout>
3) 다른 뷰와의 상대적 위치를 이용
- layout_to(Right, Left)of : 기준이 되는 뷰의 오른쪽, 왼쪽에 배치
(단, 상하의 위치를 지정하지 않으면 기본값인 Top으로 배치)
- layout_(above, below) : 기준이 되는 뷰의 위, 아래에 배치
(단, 좌우의 위치를 지정하지 않으면 기본값인 Left로 배치)
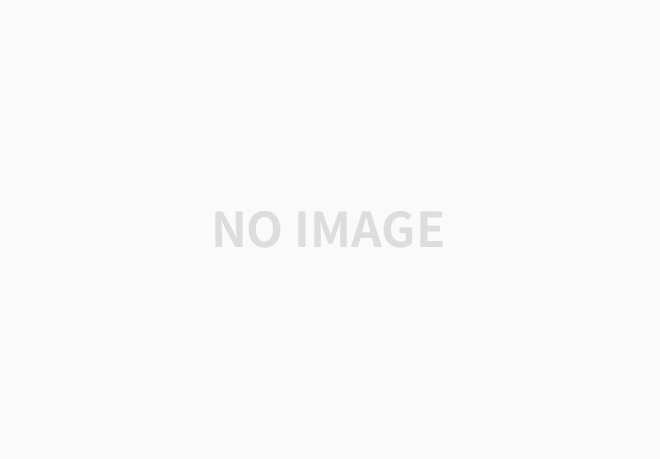
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- 1번 TextView -->
<TextView
android:id="@+id/textview01"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_centerInParent="true"
android:text="1"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 2번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_above="@id/textview01"
android:layout_toLeftOf="@id/textview01"
android:text="2"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 3번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_toRightOf="@+id/textview01"
android:layout_above="@id/textview01"
android:text="3"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 4번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_below="@id/textview01"
android:layout_toLeftOf="@id/textview01"
android:text="4"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
<!-- 5번 TextView -->
<TextView
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_below="@id/textview01"
android:layout_toRightOf="@id/textview01"
android:text="5"
android:textSize="70sp"
android:textAlignment="center"
android:background="@color/colorPrimaryDark" />
</RelativeLayout>
'Android > Layout' 카테고리의 다른 글
Layout - 03.FrameLayout (0) | 2019.04.09 |
---|---|
Layout - 01. LinearLayout (0) | 2019.04.03 |